Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
Tags
- cursor문
- 컬렉션 타입
- 생성자오버로드
- 추상메서드
- 예외미루기
- 오라클
- 다형성
- 한국건설관리시스템
- 대덕인재개발원
- 환경설정
- 자동차수리시스템
- 정수형타입
- 인터페이스
- abstract
- NestedFor
- 컬렉션프레임워크
- 자바
- 사용자예외클래스생성
- oracle
- EnhancedFor
- 객체 비교
- 예외처리
- 집합_SET
- exception
- GRANT VIEW
- 메소드오버로딩
- 어윈 사용법
- Java
- 참조형변수
- 제네릭
Archives
- Today
- Total
거니의 velog
231005_JSON 본문
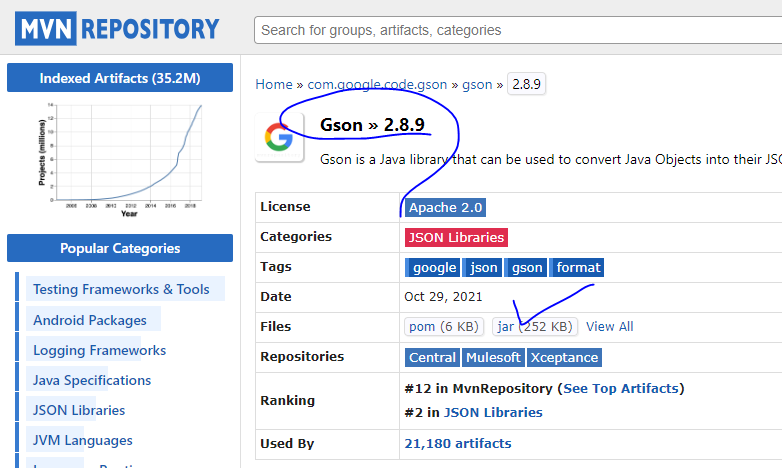
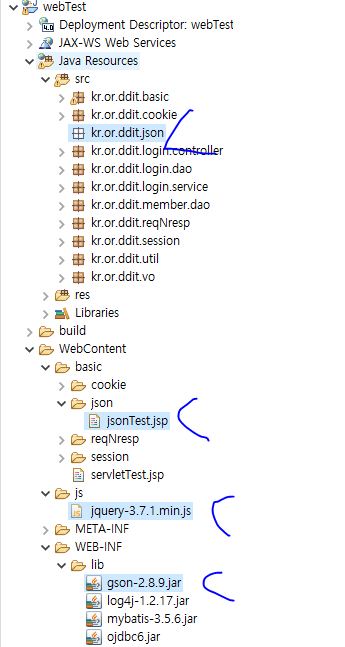
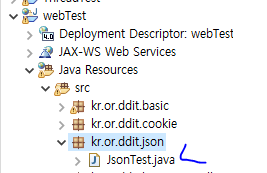
[JsonTest.java]
package kr.or.ddit.json;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.Gson;
@WebServlet("/json/jsonTest.do")
public class JsonTest extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
// 응답을 JSON데이터로 할 때의 ContentType 설정 방법
response.setContentType("application/json; charset=utf-8");
PrintWriter out = response.getWriter();
// 클라이언트가 보내온 데이터 받기
String choice = request.getParameter("choice");
// JSON처리용 객체 생성
Gson gson = new Gson();
// JSON문자열로 변환된 데이터가 저장될 변수 선언
String jsonData = null;
// 응답용 데이터를 만들어서 JSON문자열로 변환한다.
switch(choice) {
case "string" :
String str = "안녕하세요...";
jsonData = gson.toJson(str);
break;
}
// 변환된 JSON문자열을 응답으로 전송한다.
System.out.println("jsonData ==> " + jsonData);
out.write(jsonData);
response.flushBuffer();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
[jsonTest.jsp]
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>JSON 실습 1</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<script type="text/javascript">
$(function(){
// 문자열 처리
$("#strBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=string",
success : function(res){
let htmlCode = "<h3>문자열 응답 결과</h3>";
htmlCode += res;
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 배열 처리
$("#arrayBtn").on("click", function(){
});
// 객체 처리
$("#objBtn").on("click", function(){
});
// 리스트 처리
$("#listBtn").on("click", function(){
});
// Map객체 처리
$("#mapBtn").on("click", function(){
});
});
</script>
<style>
button {
margin-right: 10px;
}
</style>
</head>
<body>
<form>
<button type="button" id="strBtn">문자열</button>
<button type="button" id="arrayBtn">배열</button>
<button type="button" id="objBtn">객체</button>
<button type="button" id="listBtn">리스트</button>
<button type="button" id="mapBtn">Map객체</button>
</form>
<!-- json 응답 결과 출력 창 -->
<div id="result"></div>
</body>
</html>
- http://localhost:8090/webTest/basic/json/jsonTest.jsp
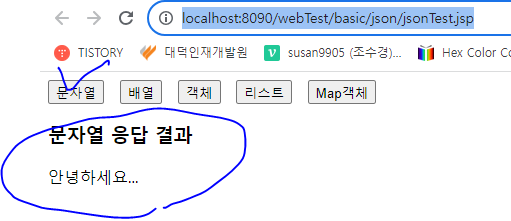
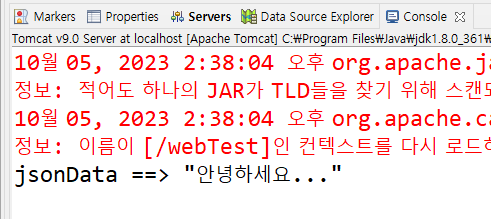
[JsonTest.java]
package kr.or.ddit.json;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.Gson;
@WebServlet("/json/jsonTest.do")
public class JsonTest extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
// 응답을 JSON데이터로 할 때의 ContentType 설정 방법
response.setContentType("application/json; charset=utf-8");
PrintWriter out = response.getWriter();
// 클라이언트가 보내온 데이터 받기
String choice = request.getParameter("choice");
// JSON처리용 객체 생성
Gson gson = new Gson();
// JSON문자열로 변환된 데이터가 저장될 변수 선언
String jsonData = null;
// 응답용 데이터를 만들어서 JSON문자열로 변환한다.
switch(choice) {
case "string" :
String str = "안녕하세요...";
jsonData = gson.toJson(str);
break;
case "array" :
int[] intArr = new int[] {10, 20, 30, 40, 50};
jsonData = gson.toJson(intArr);
break;
}
// 변환된 JSON문자열을 응답으로 전송한다.
System.out.println("jsonData ==> " + jsonData);
out.write(jsonData);
response.flushBuffer();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
[jsonTest.jsp]
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>JSON 실습 1</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<script type="text/javascript">
$(function(){
// 문자열 처리
$("#strBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=string",
success : function(res){
// res = "안녕하세요..."
let htmlCode = "<h3>문자열 응답 결과</h3>";
htmlCode += res;
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 배열 처리
$("#arrayBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=array",
success : function(res){
// res = [10, 20, 30, 40, 50];
let htmlCode = "<h3>배열 응답 결과</h3>";
htmlCode += "<ul>";
$.each(res, function(i, v){
//htmlCode += "<li>"+i+"번째 데이터</li>";
//htmlCode += "<li>"+ res[i] +"</li>";
//htmlCode += "<li>"+ v +"</li>";
//htmlCode += "<br />";
htmlCode += i + "번째 데이터 : " + v + "<br />";
});
htmlCode += "</ul>";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 객체 처리
$("#objBtn").on("click", function(){
});
// 리스트 처리
$("#listBtn").on("click", function(){
});
// Map객체 처리
$("#mapBtn").on("click", function(){
});
});
</script>
<style>
button {
margin-right: 10px;
}
</style>
</head>
<body>
<form>
<button type="button" id="strBtn">문자열</button>
<button type="button" id="arrayBtn">배열</button>
<button type="button" id="objBtn">객체</button>
<button type="button" id="listBtn">리스트</button>
<button type="button" id="mapBtn">Map객체</button>
</form>
<!-- json 응답 결과 출력 창 -->
<div id="result"></div>
</body>
</html>
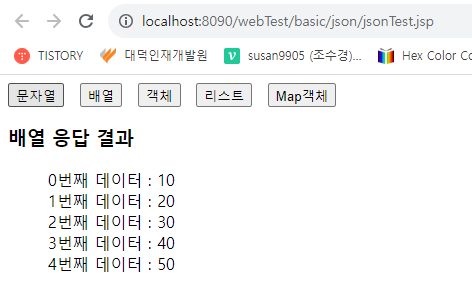

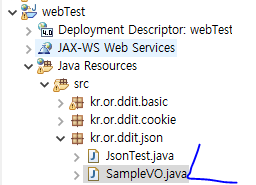
[SampleVO.java]
package kr.or.ddit.json;
public class SampleVO {
private int num;
private String name;
public SampleVO() {}
public SampleVO(int num, String name) {
this.num = num;
this.name = name;
}
public int getNum() {
return num;
}
public void setNum(int num) {
this.num = num;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "SampleVO [num=" + num + ", name=" + name + "]";
}
}
[JsonTest.java]
package kr.or.ddit.json;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.Gson;
@WebServlet("/json/jsonTest.do")
public class JsonTest extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
// 응답을 JSON데이터로 할 때의 ContentType 설정 방법
response.setContentType("application/json; charset=utf-8");
PrintWriter out = response.getWriter();
// 클라이언트가 보내온 데이터 받기
String choice = request.getParameter("choice");
// JSON처리용 객체 생성
Gson gson = new Gson();
// JSON문자열로 변환된 데이터가 저장될 변수 선언
String jsonData = null;
// 응답용 데이터를 만들어서 JSON문자열로 변환한다.
switch(choice) {
case "string" :
String str = "안녕하세요...";
jsonData = gson.toJson(str);
break;
case "array" :
int[] intArr = new int[] {10, 20, 30, 40, 50};
jsonData = gson.toJson(intArr);
break;
case "object" :
SampleVO svo = new SampleVO(1, "홍길동");
jsonData = gson.toJson(svo);
break;
}
// 변환된 JSON문자열을 응답으로 전송한다.
System.out.println("jsonData ==> " + jsonData);
out.write(jsonData);
response.flushBuffer();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
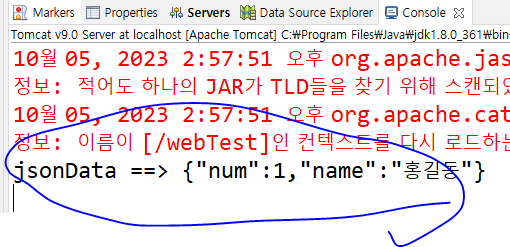
[jsonTest.jsp]
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>JSON 실습 1</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<script type="text/javascript">
$(function(){
// 문자열 처리
$("#strBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=string",
success : function(res){
// res = "안녕하세요..."
let htmlCode = "<h3>문자열 응답 결과</h3>";
htmlCode += res;
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 배열 처리
$("#arrayBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=array",
success : function(res){
// res = [10, 20, 30, 40, 50];
let htmlCode = "<h3>배열 응답 결과</h3>";
htmlCode += "<ul>";
$.each(res, function(i, v){
//htmlCode += "<li>"+i+"번째 데이터</li>";
//htmlCode += "<li>"+ res[i] +"</li>";
//htmlCode += "<li>"+ v +"</li>";
//htmlCode += "<br />";
htmlCode += i + "번째 데이터 : " + v + "<br />";
});
htmlCode += "</ul>";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 객체 처리
$("#objBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=object",
success : function(res){
// res = {"num":1,"name":"홍길동"}
let htmlCode = "<h3>객체 응답 결과</h3>";
htmlCode += "num : " + res.num + "<br />";
htmlCode += "name : " + res.name + "<br />";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 리스트 처리
$("#listBtn").on("click", function(){
});
// Map객체 처리
$("#mapBtn").on("click", function(){
});
});
</script>
<style>
button {
margin-right: 10px;
}
</style>
</head>
<body>
<form>
<button type="button" id="strBtn">문자열</button>
<button type="button" id="arrayBtn">배열</button>
<button type="button" id="objBtn">객체</button>
<button type="button" id="listBtn">리스트</button>
<button type="button" id="mapBtn">Map객체</button>
</form>
<!-- json 응답 결과 출력 창 -->
<div id="result"></div>
</body>
</html>
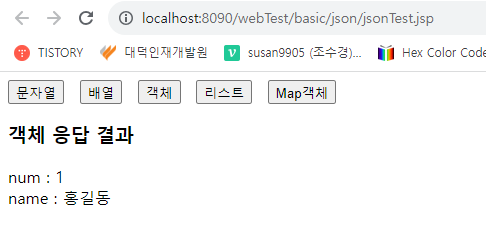
[JsonTest.java]
package kr.or.ddit.json;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.Gson;
@WebServlet("/json/jsonTest.do")
public class JsonTest extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
// 응답을 JSON데이터로 할 때의 ContentType 설정 방법
response.setContentType("application/json; charset=utf-8");
PrintWriter out = response.getWriter();
// 클라이언트가 보내온 데이터 받기
String choice = request.getParameter("choice");
// JSON처리용 객체 생성
Gson gson = new Gson();
// JSON문자열로 변환된 데이터가 저장될 변수 선언
String jsonData = null;
// 응답용 데이터를 만들어서 JSON문자열로 변환한다.
switch(choice) {
case "string" :
String str = "안녕하세요...";
jsonData = gson.toJson(str);
break;
case "array" :
int[] intArr = new int[] {10, 20, 30, 40, 50};
jsonData = gson.toJson(intArr);
break;
case "object" :
SampleVO svo = new SampleVO(1, "홍길동");
jsonData = gson.toJson(svo);
break;
case "list" :
ArrayList<SampleVO> list = new ArrayList<SampleVO>();
list.add(new SampleVO(10, "강감찬"));
list.add(new SampleVO(20, "이순신"));
list.add(new SampleVO(30, "을지문덕"));
jsonData = gson.toJson(list);
break;
}
// 변환된 JSON문자열을 응답으로 전송한다.
System.out.println("jsonData ==> " + jsonData);
out.write(jsonData);
response.flushBuffer();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}

[jsonTest.jsp]
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>JSON 실습 1</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<script type="text/javascript">
$(function(){
// 문자열 처리
$("#strBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=string",
success : function(res){
// res = "안녕하세요..."
let htmlCode = "<h3>문자열 응답 결과</h3>";
htmlCode += res;
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 배열 처리
$("#arrayBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=array",
success : function(res){
// res = [10, 20, 30, 40, 50];
let htmlCode = "<h3>배열 응답 결과</h3>";
htmlCode += "<ul>";
$.each(res, function(i, v){
//htmlCode += "<li>"+i+"번째 데이터</li>";
//htmlCode += "<li>"+ res[i] +"</li>";
//htmlCode += "<li>"+ v +"</li>";
//htmlCode += "<br />";
htmlCode += i + "번째 데이터 : " + v + "<br />";
});
htmlCode += "</ul>";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 객체 처리
$("#objBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=object",
success : function(res){
// res = {"num":1,"name":"홍길동"}
let htmlCode = "<h3>객체 응답 결과</h3>";
htmlCode += "num : " + res.num + "<br />";
htmlCode += "name : " + res.name + "<br />";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 리스트 처리
$("#listBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=list",
success : function(res){
/*
res = [
{"num":10,"name":"강감찬"},
{"num":20,"name":"이순신"},
{"num":30,"name":"을지문덕"}
];
*/
let htmlCode = "<h3>List 응답 결과</h3>";
$.each(res, function(i, v){
htmlCode += i + "번째 자료<br />";
htmlCode += "num : " + v.num + "<br />";
htmlCode += "name : " + v.name + "<hr />";
});
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// Map객체 처리
$("#mapBtn").on("click", function(){
});
});
</script>
<style>
button {
margin-right: 10px;
}
</style>
</head>
<body>
<form>
<button type="button" id="strBtn">문자열</button>
<button type="button" id="arrayBtn">배열</button>
<button type="button" id="objBtn">객체</button>
<button type="button" id="listBtn">리스트</button>
<button type="button" id="mapBtn">Map객체</button>
</form>
<!-- json 응답 결과 출력 창 -->
<div id="result"></div>
</body>
</html>
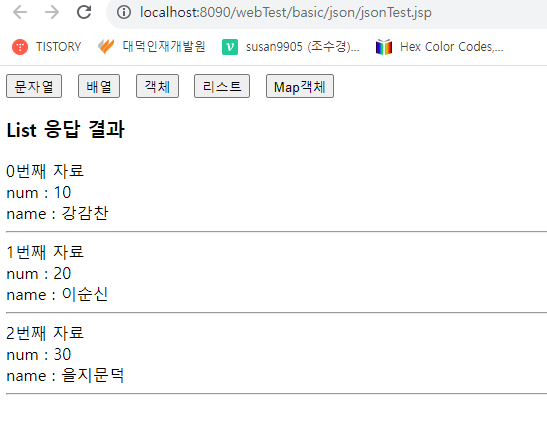
[JsonTest.java]
package kr.or.ddit.json;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.HashMap;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.Gson;
@WebServlet("/json/jsonTest.do")
public class JsonTest extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
// 응답을 JSON데이터로 할 때의 ContentType 설정 방법
response.setContentType("application/json; charset=utf-8");
PrintWriter out = response.getWriter();
// 클라이언트가 보내온 데이터 받기
String choice = request.getParameter("choice");
// JSON처리용 객체 생성
Gson gson = new Gson();
// JSON문자열로 변환된 데이터가 저장될 변수 선언
String jsonData = null;
// 응답용 데이터를 만들어서 JSON문자열로 변환한다.
switch(choice) {
case "string" :
String str = "안녕하세요...";
jsonData = gson.toJson(str);
break;
case "array" :
int[] intArr = new int[] {10, 20, 30, 40, 50};
jsonData = gson.toJson(intArr);
break;
case "object" :
SampleVO svo = new SampleVO(1, "홍길동");
jsonData = gson.toJson(svo);
break;
case "list" :
ArrayList<SampleVO> list = new ArrayList<SampleVO>();
list.add(new SampleVO(10, "강감찬"));
list.add(new SampleVO(20, "이순신"));
list.add(new SampleVO(30, "을지문덕"));
jsonData = gson.toJson(list);
break;
case "map" :
HashMap<String, String> map = new HashMap<String, String>();
map.put("name", "성춘향");
map.put("tel", "010-1234-5678");
map.put("addr", "대전시 중구 오류동");
jsonData = gson.toJson(map);
break;
}
// 변환된 JSON문자열을 응답으로 전송한다.
System.out.println("jsonData ==> " + jsonData);
out.write(jsonData);
response.flushBuffer();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
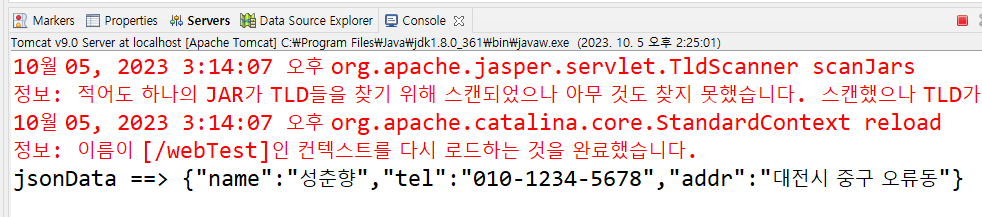
[jsonTest.jsp]
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>JSON 실습 1</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<script type="text/javascript">
$(function(){
// 문자열 처리
$("#strBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=string",
success : function(res){
// res = "안녕하세요..."
let htmlCode = "<h3>문자열 응답 결과</h3>";
htmlCode += res;
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 배열 처리
$("#arrayBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=array",
success : function(res){
// res = [10, 20, 30, 40, 50];
let htmlCode = "<h3>배열 응답 결과</h3>";
htmlCode += "<ul>";
$.each(res, function(i, v){
//htmlCode += "<li>"+i+"번째 데이터</li>";
//htmlCode += "<li>"+ res[i] +"</li>";
//htmlCode += "<li>"+ v +"</li>";
//htmlCode += "<br />";
htmlCode += i + "번째 데이터 : " + v + "<br />";
});
htmlCode += "</ul>";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 객체 처리
$("#objBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=object",
success : function(res){
// res = {"num":1,"name":"홍길동"}
let htmlCode = "<h3>객체 응답 결과</h3>";
htmlCode += "num : " + res.num + "<br />";
htmlCode += "name : " + res.name + "<br />";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// 리스트 처리
$("#listBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=list",
success : function(res){
/*
res = [
{"num":10,"name":"강감찬"},
{"num":20,"name":"이순신"},
{"num":30,"name":"을지문덕"}
];
*/
let htmlCode = "<h3>List 응답 결과</h3>";
$.each(res, function(i, v){
htmlCode += i + "번째 자료<br />";
htmlCode += "num : " + v.num + "<br />";
htmlCode += "name : " + v.name + "<hr />";
});
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
// Map객체 처리하기
$("#mapBtn").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/json/jsonTest.do",
type : "post",
data : "choice=map",
success : function(res){
/*
res = {
"name":"성춘향",
"tel":"010-1234-5678",
"addr":"대전시 중구 오류동"
}
*/
let htmlCode = "<h3>Map 응답 결과</h3>";
/*
htmlCode += "name : " + res.name + "<br />";
htmlCode += "tel : " + res.tel + "<br />";
htmlCode += "addr : " + res.addr + "<hr />";
*/
$.each(res, function(i, v){
htmlCode += i + " : " + v + "<br />";
});
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
});
</script>
<style>
button {
margin-right: 10px;
}
</style>
</head>
<body>
<form>
<button type="button" id="strBtn">문자열</button>
<button type="button" id="arrayBtn">배열</button>
<button type="button" id="objBtn">객체</button>
<button type="button" id="listBtn">리스트</button>
<button type="button" id="mapBtn">Map객체</button>
</form>
<!-- json 응답 결과 출력 창 -->
<div id="result"></div>
</body>
</html>

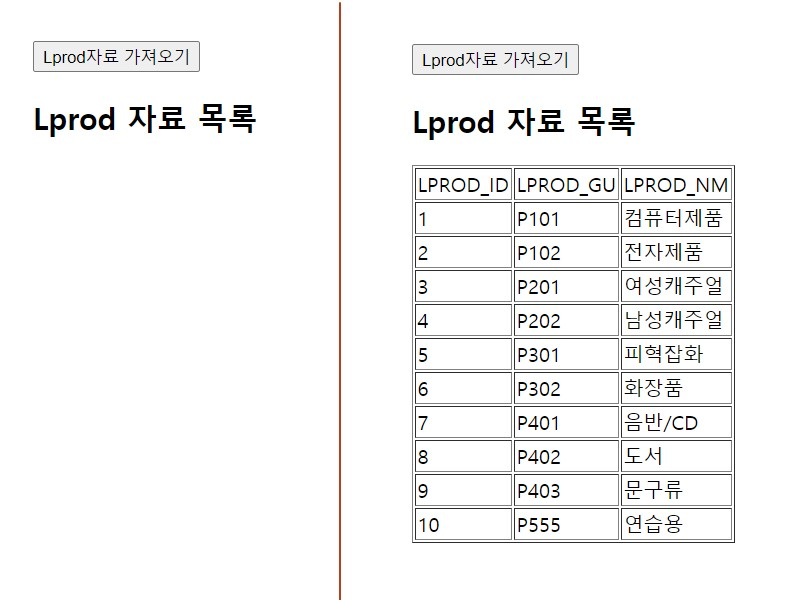

[mybatis-config.xml]
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org/DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- DB연결 정보가 저장된 properties파일에 대한 정보를 설정한다. -->
<properties resource="kr/or/ddit/mybatis/config/dbinfo.properties" />
<!-- MyBatis 설정과 관련된 기본 setting 설정 -->
<settings>
<!-- 데이터가 null로 전달되었으면 빈칸으로 인식하지 말고 null로 인식하라 -->
<setting name="jdbcTypeForNull" value="NULL" />
</settings>
<typeAliases>
<typeAlias type="kr.or.ddit.vo.MemberVO" alias="memVo" />
<typeAlias type="kr.or.ddit.vo.LprodVO" alias="lprodVo" />
</typeAliases>
<!-- DB연결 설정 -->
<environments default="oracleDev">
<environment id="oracleDev">
<!-- 오라클 -->
<transactionManager type="JDBC" />
<dataSource type="POOLED">
<property name="driver" value="${driver}" />
<property name="url" value="${url}" />
<property name="username" value="${user}" />
<property name="password" value="${pass}" />
</dataSource>
</environment>
<!-- <environment id="m1">
MySql
</environment> -->
</environments>
<mappers>
<mapper resource="kr/or/ddit/mybatis/mappers/member-mapper.xml" />
<mapper resource="kr/or/ddit/mybatis/mappers/lprod-mapper.xml" />
</mappers>
</configuration>
[lprod-mapper.xml]
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org/DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="lprod">
<select id="getAllProd" resultType="lprodVo">
select * from lprod
</select>
</mapper>
[LprodVO.java]
package kr.or.ddit.vo;
public class LprodVO {
private int lprod_id;
private String lprod_gu;
private String lprod_nm;
public LprodVO() {}
public LprodVO(int lprod_id, String lprod_gu, String lprod_nm) {
this.lprod_id = lprod_id;
this.lprod_gu = lprod_gu;
this.lprod_nm = lprod_nm;
}
public int getLprod_id() {
return lprod_id;
}
public void setLprod_id(int lprod_id) {
this.lprod_id = lprod_id;
}
public String getLprod_gu() {
return lprod_gu;
}
public void setLprod_gu(String lprod_gu) {
this.lprod_gu = lprod_gu;
}
public String getLprod_nm() {
return lprod_nm;
}
public void setLprod_nm(String lprod_nm) {
this.lprod_nm = lprod_nm;
}
@Override
public String toString() {
return "LprodVO [lprod_id=" + lprod_id + ", lprod_gu=" + lprod_gu + ", lprod_nm=" + lprod_nm + "]";
}
}
[LprodDaoImpl.java]
package kr.or.ddit.json;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import kr.or.ddit.util.MyBatisUtil;
import kr.or.ddit.vo.LprodVO;
public class LprodDaoImpl {
// 싱글턴 패턴
private static LprodDaoImpl dao;
private LprodDaoImpl() {}
public static LprodDaoImpl getInstance() {
if(dao == null) dao = new LprodDaoImpl();
return dao;
}
public List<LprodVO> getAllProd() {
SqlSession session = MyBatisUtil.getSqlSession();
List<LprodVO> lprodList = null;
try {
lprodList = session.selectList("lprod.getAllProd");
} catch (Exception e) {
e.printStackTrace();
} finally {
if(session != null) session.close();
}
return lprodList;
}
}
[LprodList.java]
package kr.or.ddit.json;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.Gson;
import kr.or.ddit.vo.LprodVO;
@WebServlet("/lprod/lprodList.do")
public class LprodList extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
response.setContentType("application/json; charset=utf-8");
PrintWriter out = response.getWriter();
LprodDaoImpl dao = LprodDaoImpl.getInstance();
List<LprodVO> lprodList = dao.getAllProd();
Gson gson = new Gson();
String jsonData = gson.toJson(lprodList);
out.write(jsonData);
response.flushBuffer();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
[lprodMain.jsp]
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>LprodMain</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js"></script>
<script type="text/javascript">
$(function(){
$("#lprodBtn1").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/lprod/lprodList.do",
type : "post",
success : function(res){
var htmlCode = "<table class='table table-dark table-hover'>";
htmlCode += "<tr><th>LPROD_ID</th><th>LPROD_GU</th><th>LPROD_NM</th></tr>";
$.each(res, function(i, v){
htmlCode += "<tr><td>"+ v.lprod_id +"</td>";
htmlCode += "<td>"+ v.lprod_gu +"</td>";
htmlCode += "<td>"+ v.lprod_nm +"</td></tr>";
});
htmlCode += "</table>";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
});
</script>
</head>
<body>
<button id="lprodBtn1" class="btn btn-primary" type="button">Lprod 자료 가져오기(ajax)</button>
<button id="lprodBtn2" class="btn btn-primary" type="button">Lprod 자료 가져오기(동기방식)</button>
<hr />
<h2>Lprod 자료 목록</h2>
<div id="result"></div>
</body>
</html>
- http://localhost:8090/webTest/basic/json/lprodMain.jsp
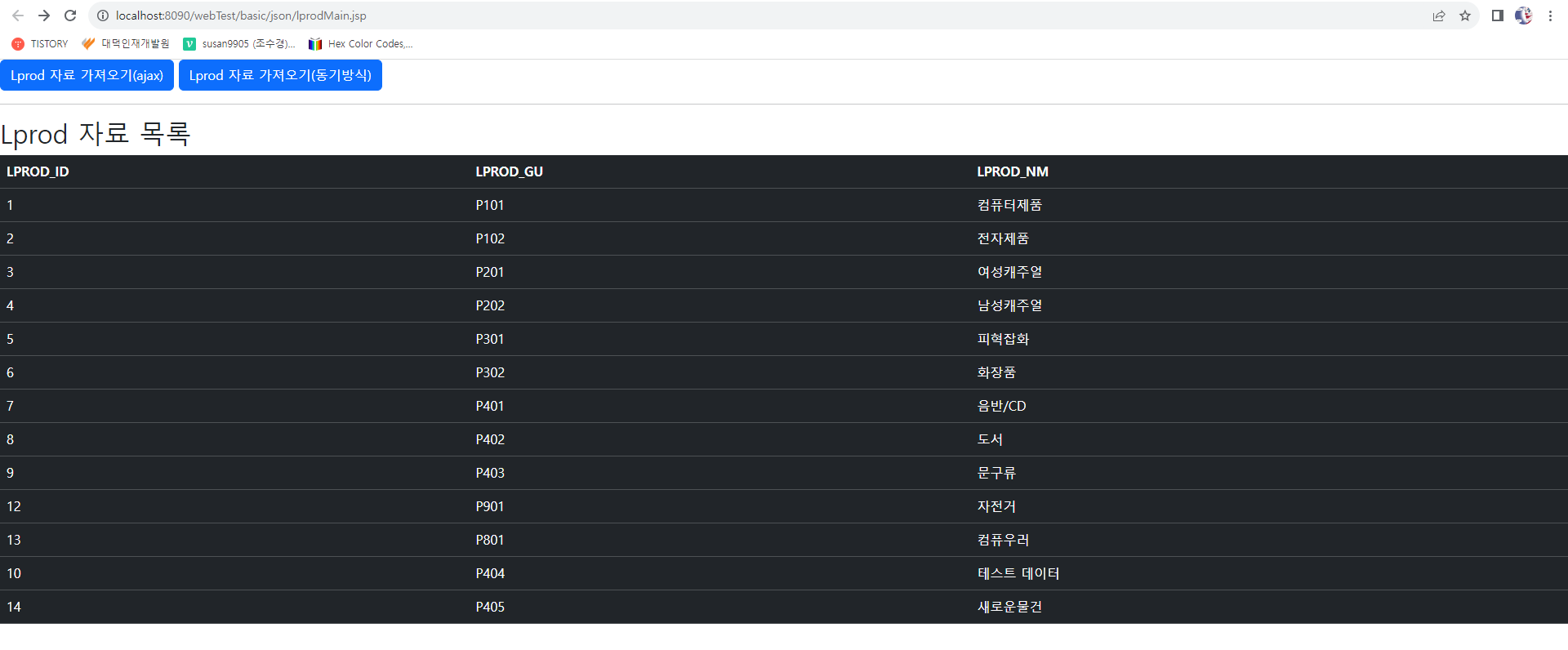
[동기 방식으로 처리하기]
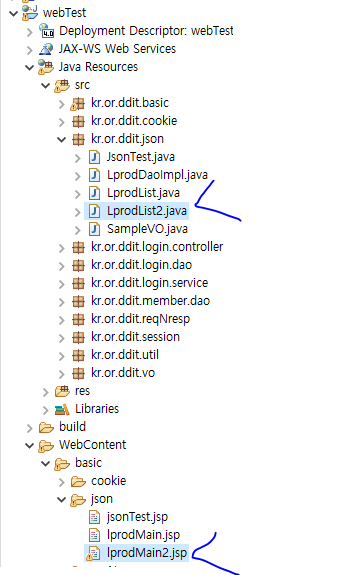
[LprodList2.java]
package kr.or.ddit.json;
import java.io.IOException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import kr.or.ddit.vo.LprodVO;
@WebServlet("/lprod/lprodList2.do")
public class LprodList2 extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
LprodDaoImpl dao = LprodDaoImpl.getInstance();
List<LprodVO> lprodList = dao.getAllProd();
request.setAttribute("lprodList", lprodList);
request.getRequestDispatcher("/basic/json/lprodMain2.jsp").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
[lprodMain2.jsp]
<%@page import="kr.or.ddit.vo.LprodVO"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>LprodMain2</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<%
// Controller(서블릿) 보낸 데이터 받기
List<LprodVO> lprodList = (List<LprodVO>) request.getAttribute("lprodList");
%>
<h3>Lprod 자료 목록</h3>
<table class='table table-dark table-hover'>
<tr>
<th>LPROD_ID</th>
<th>LPROD_GU</th>
<th>LPROD_NM</th>
</tr>
<%
for(LprodVO lvo : lprodList) {
%>
<tr>
<td><%= lvo.getLprod_id() %></td>
<td><%= lvo.getLprod_gu() %></td>
<td><%= lvo.getLprod_nm() %></td>
</tr>
<%
}
%>
</table>
</body>
</html>
- http://localhost:8090/webTest/lprod/lprodList2.do
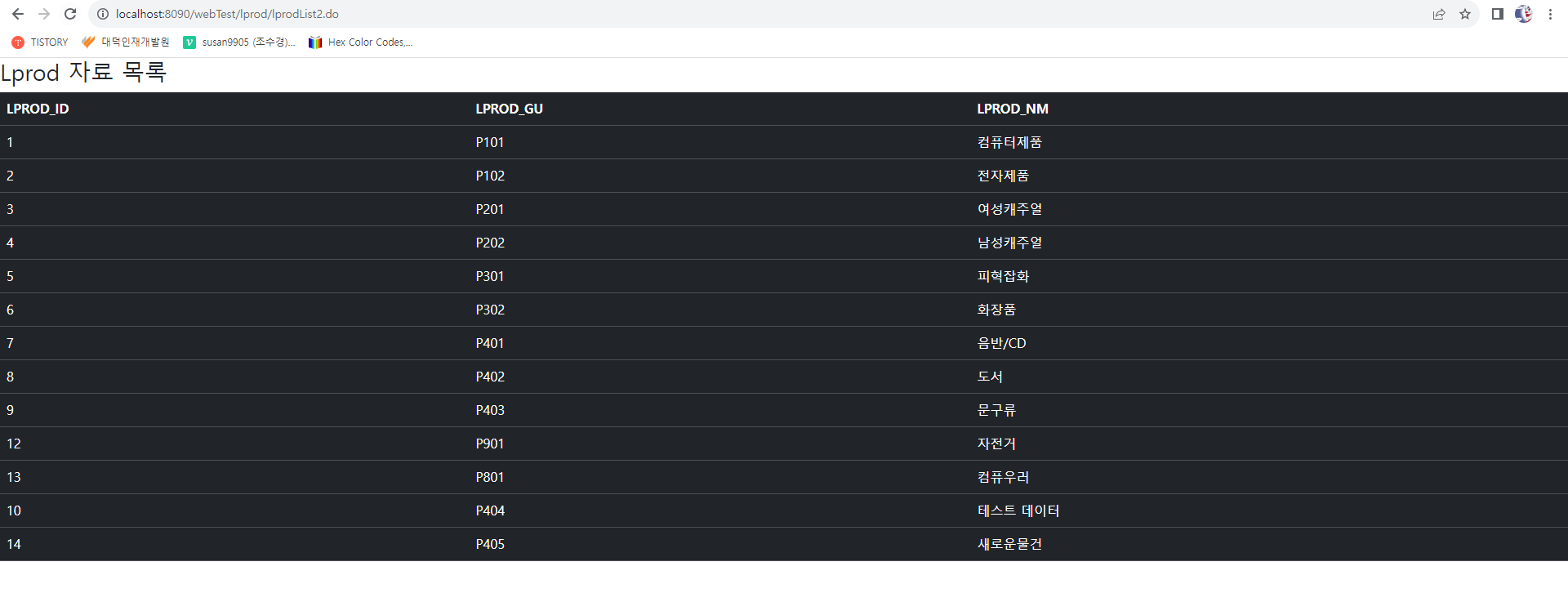
[lprodMain.jsp]
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>LprodMain</title>
<script type="text/javascript" src="<%= request.getContextPath() %>/js/jquery-3.7.1.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js"></script>
<script type="text/javascript">
$(function(){
$("#lprodBtn1").on("click", function(){
$.ajax({
url : "<%= request.getContextPath() %>/lprod/lprodList.do",
type : "post",
success : function(res){
var htmlCode = "<table class='table table-dark table-hover'>";
$.each(res, function(i, v){
htmlCode += "<tr><td>"+ v.lprod_id +"</td>";
htmlCode += "<td>"+ v.lprod_gu +"</td>";
htmlCode += "<td>"+ v.lprod_nm +"</td></tr>";
});
htmlCode += "</table>";
$("#result").html(htmlCode);
},
error : function(xhr){
alert("상태 : " + xhr.status);
},
dataType : "json"
});
});
$("#lprodBtn2").on("click", function(){
location.href="<%=request.getContextPath()%>/lprod/lprodList2.do";
});
});
</script>
</head>
<body>
<button id="lprodBtn1" class="btn btn-primary" type="button">Lprod 자료 가져오기(ajax)</button>
<button id="lprodBtn2" class="btn btn-primary" type="button">Lprod 자료 가져오기(동기방식)</button>
<hr />
<h2>Lprod 자료 목록</h2>
<div id="result"></div>
</body>
</html>
- http://localhost:8090/webTest/basic/json/lprodMain.jsp
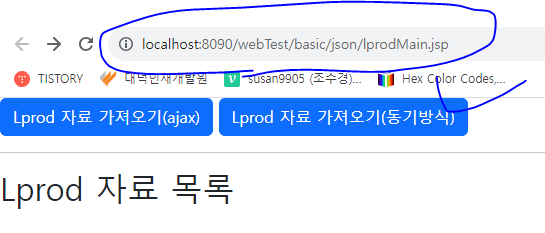
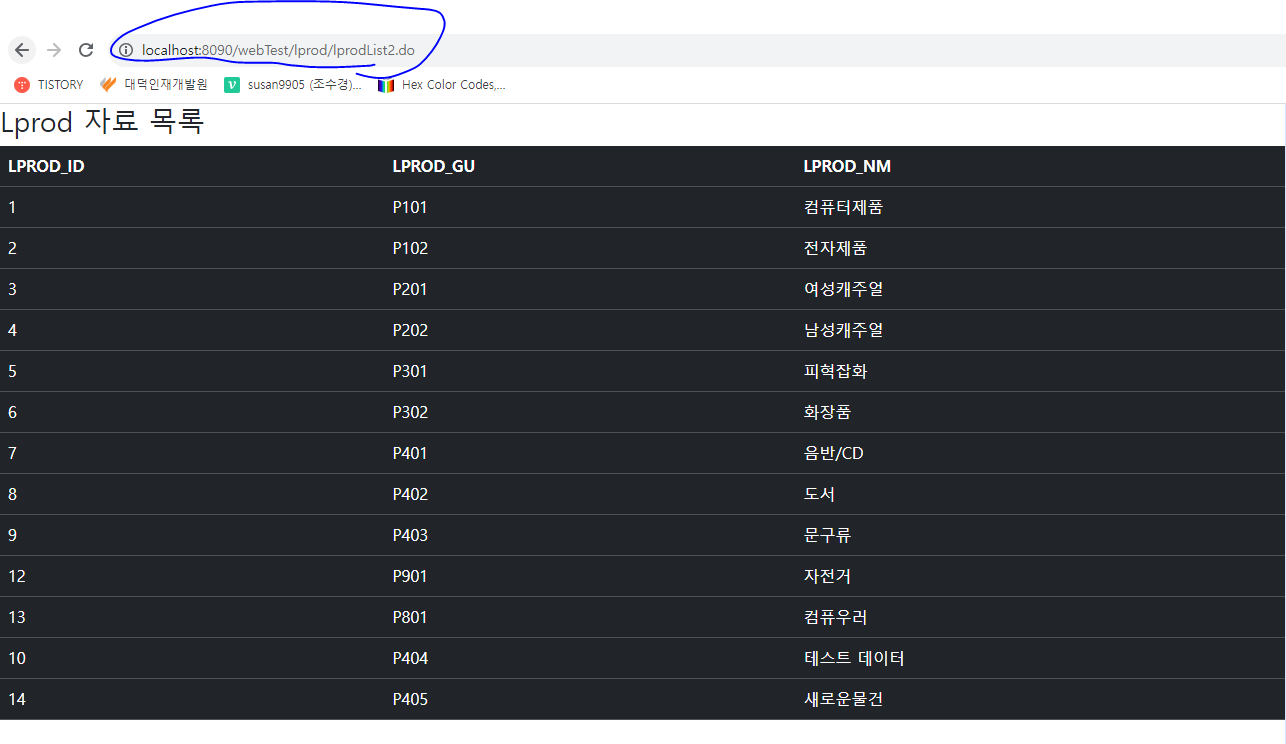
'대덕인재개발원_자바기반 애플리케이션' 카테고리의 다른 글
231005_고급자바과제_회원정보관리 (1) | 2023.10.07 |
---|---|
231005_File 업로드, 다운로드 (0) | 2023.10.05 |
231004_유스케이스 다이어그램 작성 (0) | 2023.10.04 |
231004_Servlet 5 (0) | 2023.10.02 |
231002_Servlet 4 (0) | 2023.09.27 |